Last active
October 1, 2020 19:42
-
-
Save nineonefive/801a957a6cee26ed9870eff1f8a45f3d to your computer and use it in GitHub Desktop.
Script that can visualize how images (like banners or sprays) would look in Minecraft 1.8
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Original image (1920x1080):
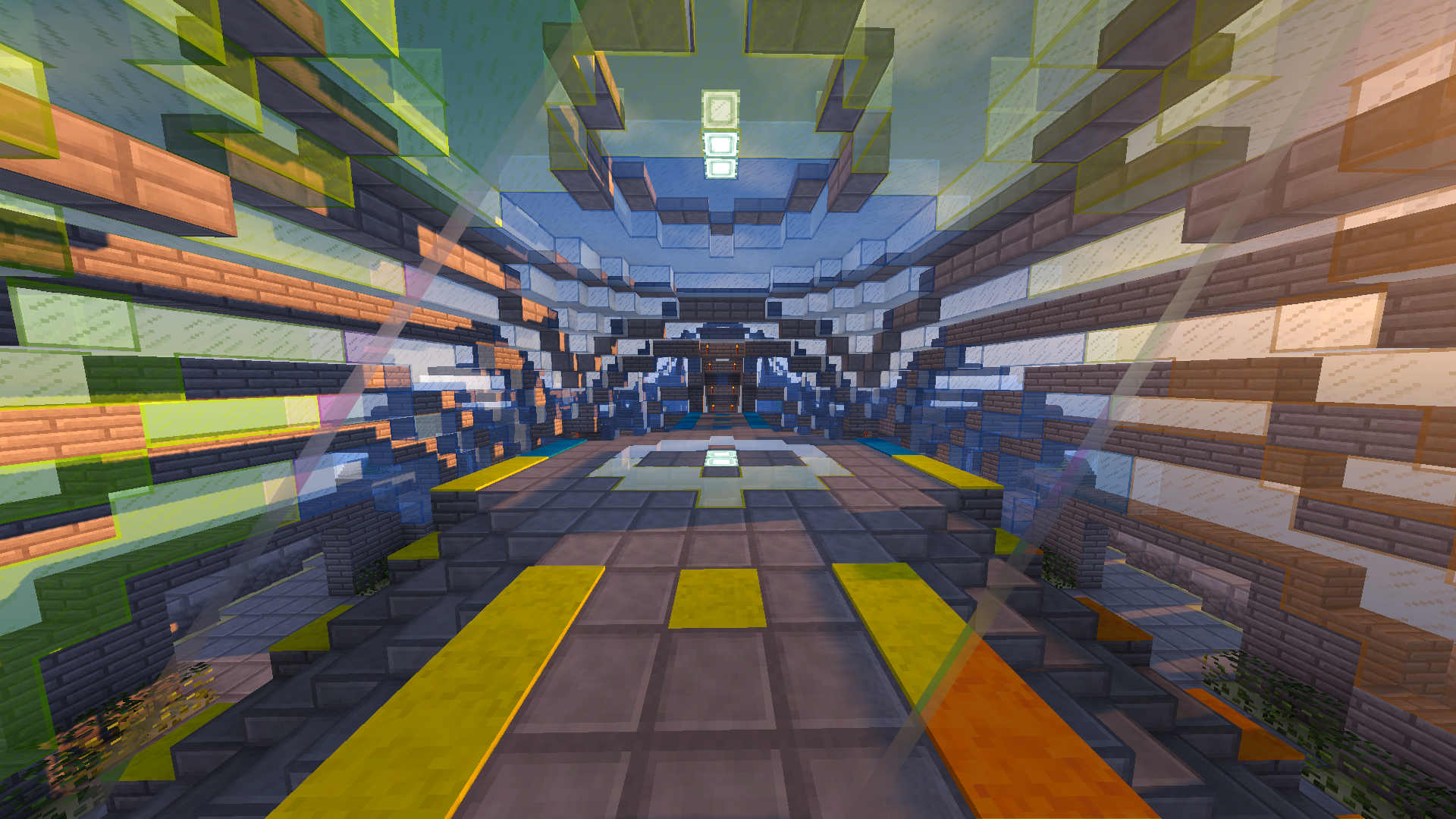
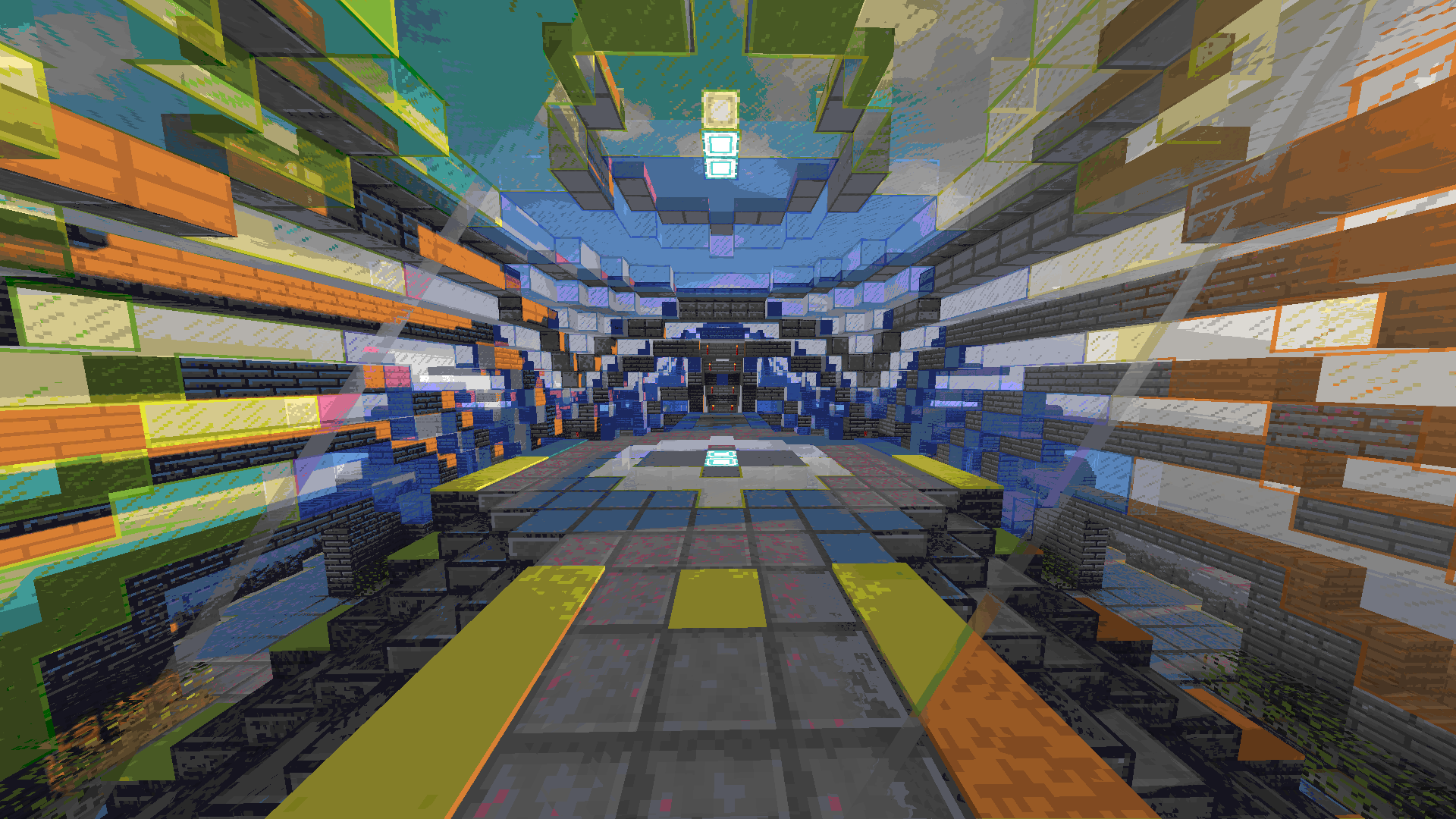
In MC 1.8: