Last active
September 9, 2021 02:32
-
-
Save mrdotkg/15c678c64773491184e0042619eec5d4 to your computer and use it in GitHub Desktop.
Shell into ec2 through ssm
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#!/usr/bin/python3 | |
"""Usage: | |
sh_ec2.py [--instance=<instance_id>] | |
sh_ec2.py -h | --help | |
sh_ec2.py --version | |
Options: | |
-h --help Show this screen | |
-i --instance=<instance_id> EC2 instance id | |
--version Show version | |
""" | |
import subprocess | |
import inquirer | |
import boto3 | |
from docopt import docopt | |
arguments = docopt(__doc__, version='instance connector - shec2.py 1.0') | |
config = { | |
'Instance' : arguments['--instance'], | |
} | |
# Find out ec2 instance id | |
if config['Instance'] is None: | |
ec2 = boto3.resource('ec2') | |
instance_choices = [] | |
for instance in ec2.instances.all(): | |
instance_id = '' | |
instance_name ='' | |
# if { Key: "Shell", Value: "SSM"} in instance.tags: | |
for tag in instance.tags: | |
if tag['Key'] == 'Name': | |
instance_name=tag['Value'] | |
instance_id = instance.id | |
instance_choices.append( instance_name + " " + instance_id) | |
if instance_choices: | |
questions = [ | |
inquirer.List('instance', | |
message="Which Instance?", | |
choices=instance_choices | |
) | |
] | |
answers = inquirer.prompt(questions) | |
selected_instance_id = answers['instance'].split(' ')[1] | |
config['Instance'] = selected_instance_id | |
else: | |
exit('No instance is running in default aws account') | |
# Provide shell into the EC2 instance | |
subprocess.run(['aws', 'ssm', 'start-session', '--target', config['Instance']]) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
How to run this script
Output
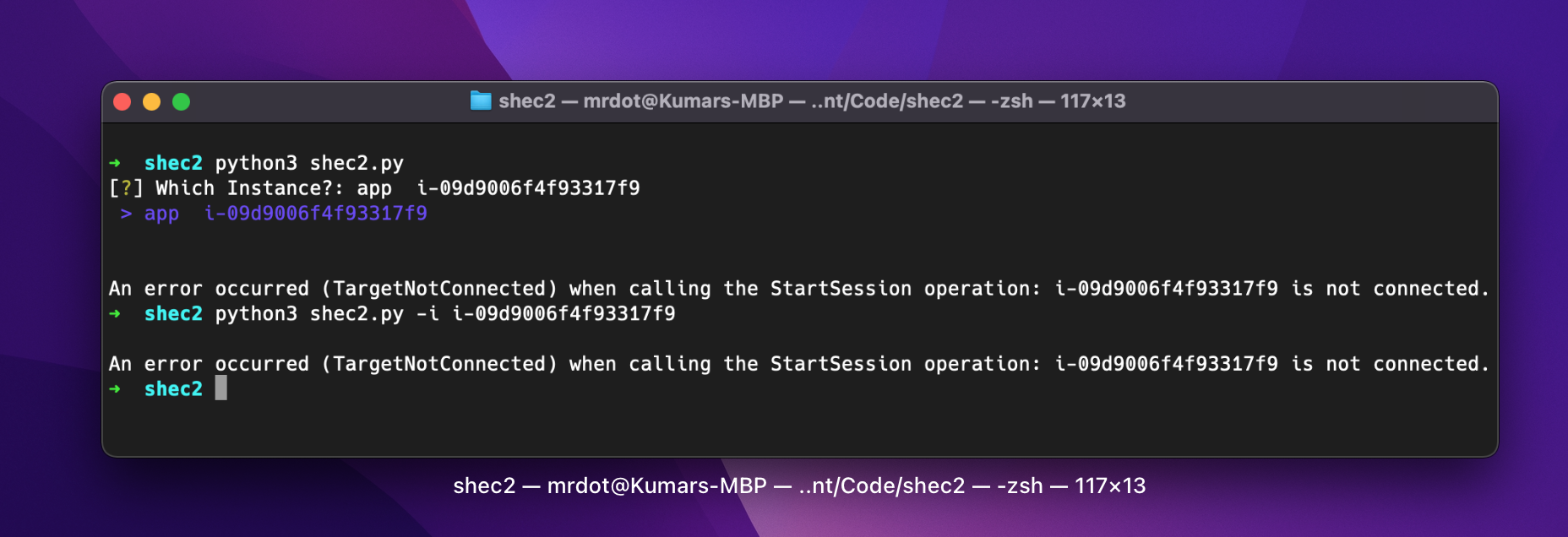