-
-
Save deepak3081996/ca77083f3780280c38a039add2b4a290 to your computer and use it in GitHub Desktop.
from selenium import webdriver | |
from selenium.webdriver.support.ui import WebDriverWait | |
from selenium.webdriver.support import expected_conditions as EC | |
from selenium.webdriver.common.by import By | |
from selenium.webdriver.common.keys import Keys | |
def message(to,message=''): | |
"""this a simple function to send a whatsapp message to your friends | |
and group using python and selenium an automated tool to parse the HTML | |
content and to change the properties. | |
paramters: | |
to - enter a name from your contacts it can be friend's name or a group's title. | |
message - message to be deliever""" | |
d = webdriver.Chrome(r'F:/chrome driver/chromedriver.exe') # directory to chromedriver | |
d.get('https://web.whatsapp.com/') # URL to open whatsapp web | |
wait = WebDriverWait(driver = d, timeout = 900) # inscrease or decrease the timeout according to your net connection | |
message += '\nthis is a system generated message' # additional text to with your message to identify that it is send via software | |
name_argument = f'//span[contains(@title,\'{to}\')]' # HTML parse code to identify your reciever | |
title = wait.until(EC.presence_of_element_located((By.XPATH,name_argument))) | |
title.click() # to open the receiver messages page in the browser | |
# many a times class name or other HTML properties changes so keep a track of current class name for input box by using inspect elements | |
input_path = '//div[@class="pluggable-input-body copyable-text selectable-text"][@dir="auto"][@data-tab="1"]' | |
box = wait.until(EC.presence_of_element_located((By.XPATH,input_path))) | |
box.send_keys(message + Keys.ENTER) # send your message followed by an Enter |
Hello Can you automate whatsapp for me .
Please contact for WhatsApp automation.
Pls Whatsapp +91 9785201393
Hey I tried the above script and works like charm what if I want to send multiple people at the same time can you guide with that and also when the name has space it seems not detecting can you please check its my early days in learning python
This is slightly improved version of Solomon code, it will keep you logged in.
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
options = webdriver.ChromeOptions();
options.add_argument('--user-data-dir=./User_Data')
driver = webdriver.Chrome(chrome_options=options)
driver.get('https://web.whatsapp.com/')
name = input('Enter the name of user or group : ')
msg = input('Enter your message : ')
count = int(input('Enter the count : '))
wait = WebDriverWait(driver = driver, timeout = 900)
user = driver.find_element_by_xpath('//span[@title = "{}"]'.format(name))
user.click()
msg_box = driver.find_element_by_class_name('_2S1VP')
#msg += '\n this is a system generated message'
for i in range(count):
msg_box.send_keys(msg)
button = driver.find_element_by_class_name('_35EW6')
button.click()
wait = WebDriverWait(driver = driver, timeout = 600)
driver.close()
I tried the code provided by the @webeyez and got stuck on the following error
NoSuchElementException: Message: no such element: Unable to locate element: {"method":"xpath","selector":"//span[@title = "vinee"]"} (Session info: chrome=81.0.4044.138)
import pywhatkit as kit
import datetime
import sys
ask = input('''do you want to send a scheduled message (y/n)
choose n if you want to send message now : ''')
while True:
if ask == "n":
no = input("enter the phone no with country code :")
mess = input("enter your message: ")
h = datetime.datetime.now().hour
m = datetime.datetime.now().minute + 1
if no == "exit":
sys.exit()
else:
kit.sendwhatmsg(no, mess , h, m)
print("message sent")
elif ask == "y":
no = input("enter the phone no with country code :")
mess = input("enter your message: ")
print("enter the time you want to send the message|")
h = int(input("hours: "))
m = int(input("minutes: "))
if no == "exit":
sys.exit()
else:
kit.sendwhatmsg(no, mess , h, m)
print("message sent")
elif ask == "s":
pywhatkit.headless_pyk.sendwhatmsg(...)
else:
print("give a proper input (y/n) only")
How to implement this code, anyone suggest please iam trying to message to multiple whatsapp group members in a group
Hi. I need to send whatsapp messages(with and without attachments both) to unsaved contacts. Can you please share how can we do that?
I do not have much knowledge on this. I took code from one repository it was working fine few days ago but now the "Send" is not working. After lot of google search I understood looks like whtasapp might have done some html version changes so the the code which i have is not compatible with it. I tried to get the new Xpath for send button but no luck.
here is the code: I added print messages to trace the failure and I am getting this user defined message: Fail during click on send button.
The commented lines are the new Xpaths I picked from "inspect".
def send_message_to_unsaved_contact(number,msg,count):
# Reference : https://faq.whatsapp.com/en/android/26000030/
print("In send_message_to_unsaved_contact method")
parms = {'phone': str(number), 'text': str(msg)}
end = urllib.parse.urlencode(parms)
final_url = Link + 'send?' + end
print(final_url)
driver.get(final_url)
WebDriverWait(driver, 300).until(EC.presence_of_element_located((By.XPATH, '//div[@title = "Menu"]')))
print("Page loaded successfully.")
for retry in range(3):
try:
sleep(5)
wait.until(EC.presence_of_element_located((By.XPATH, '//*[@id="main"]/footer/div[1]/div[3]/button'))).click()
# wait.until(EC.presence_of_element_located((By.XPATH, '//*[@id="main"]/footer/div[1]/div[2]/div/div[2]/button'))).click()
break
except Exception as e:
print("Fail during click on send button.")
if retry==2:return
msg_box = driver.find_element_by_xpath('//*[@id="main"]/footer/div[1]/div[2]/div/div[2]')
for index in range(count-1):
msg_box.send_keys(msg)
driver.find_element_by_xpath('//*[@id="main"]/footer/div[1]/div[3]/button').click()
# driver.find_element_by_xpath('//*[@id="main"]/footer/div[1]/div[2]/div/div[2]/button').click()
print("Message sent successfully to: ",number)
Thanks in advance for support.
This is slightly improved version of Solomon code, it will keep you logged in.
from selenium import webdriver from selenium.webdriver.support.ui import WebDriverWait options = webdriver.ChromeOptions(); options.add_argument('--user-data-dir=./User_Data') driver = webdriver.Chrome(chrome_options=options) driver.get('https://web.whatsapp.com/') name = input('Enter the name of user or group : ') msg = input('Enter your message : ') count = int(input('Enter the count : ')) wait = WebDriverWait(driver = driver, timeout = 900) user = driver.find_element_by_xpath('//span[@title = "{}"]'.format(name)) user.click() msg_box = driver.find_element_by_class_name('_2S1VP') #msg += '\n this is a system generated message' for i in range(count): msg_box.send_keys(msg) button = driver.find_element_by_class_name('_35EW6') button.click() wait = WebDriverWait(driver = driver, timeout = 600) driver.close()
i tried this and getting this error, can you help
Warning (from warnings module):
File "D:\College\Python\Next\try.py", line 6
driver = webdriver.Chrome(chrome_options=options)
DeprecationWarning: use options instead of chrome_options
Traceback (most recent call last):
File "D:\PYTHON\lib\site-packages\selenium\webdriver\common\service.py", line 71, in start
self.process = subprocess.Popen(cmd, env=self.env,
File "D:\PYTHON\lib\subprocess.py", line 969, in init
self._execute_child(args, executable, preexec_fn, close_fds,
File "D:\PYTHON\lib\subprocess.py", line 1438, in _execute_child
hp, ht, pid, tid = _winapi.CreateProcess(executable, args,
FileNotFoundError: [WinError 2] The system cannot find the file specified
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "D:\College\Python\Next\try.py", line 6, in
driver = webdriver.Chrome(chrome_options=options)
File "D:\PYTHON\lib\site-packages\selenium\webdriver\chrome\webdriver.py", line 69, in init
super().init(DesiredCapabilities.CHROME['browserName'], "goog",
File "D:\PYTHON\lib\site-packages\selenium\webdriver\chromium\webdriver.py", line 89, in init
self.service.start()
File "D:\PYTHON\lib\site-packages\selenium\webdriver\common\service.py", line 81, in start
raise WebDriverException(
selenium.common.exceptions.WebDriverException: Message: 'chromedriver' executable needs to be in PATH. Please see https://chromedriver.chromium.org/home
This is much better and user-friendly too...check this out
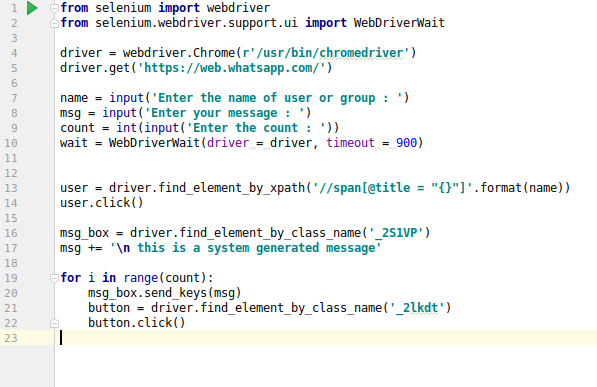