Created
June 19, 2022 09:55
-
-
Save ArthurZhou/98a3cbaa092bbd864cfa5d5c19195239 to your computer and use it in GitHub Desktop.
A simple login page
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
<!DOCTYPE html> | |
<html lang="en"> | |
<head> | |
<meta charset="UTF-8"> | |
<meta content="IE=edge" http-equiv="X-UA-Compatible"> | |
<meta content="width=device-width, initial-scale=1.0" name="viewport"> | |
<title>Login</title> | |
<style> | |
@keyframes fadeInAnimation { | |
0% { | |
opacity: 0; | |
backdrop-filter: blur(0px); | |
display: none; | |
} | |
100% { | |
opacity: 1; | |
backdrop-filter: blur(10px); | |
display: block; | |
} | |
} | |
* { | |
margin: 0; | |
padding: 0; | |
font-family: Arial, sans-serif; | |
} | |
body { | |
height: 100vh; | |
background: url(image/bg.jpg) no-repeat; | |
background-size: cover; | |
display: flex; | |
justify-content: center; | |
align-items: center; | |
} | |
.box { | |
width: 350px; | |
height: 380px; | |
border-top: 2px solid rgba(195, 195, 195, 0.3); | |
border-left: 2px solid rgba(195, 195, 195, 0.3); | |
border-bottom: 2px solid rgba(195, 195, 195, 0.3); | |
border-right: 0; | |
animation: fadeInAnimation ease 1.0s; | |
animation-iteration-count: 1; | |
animation-fill-mode: forwards; | |
background: rgba(16, 16, 16, 0.4); | |
display: flex; | |
flex-direction: column; | |
justify-content: center; | |
align-items: center; | |
border-top-left-radius: 20px; | |
border-bottom-left-radius: 20px; | |
} | |
.info_box { | |
width: 100px; | |
height: 380px; | |
margin-left: 10px; | |
border-top: 2px solid rgba(195, 195, 195, 0.3); | |
border-left: 0; | |
border-bottom: 2px solid rgba(195, 195, 195, 0.3); | |
border-right: 2px solid rgba(195, 195, 195, 0.3); | |
animation: fadeInAnimation ease 2.0s; | |
animation-iteration-count: 1; | |
animation-fill-mode: forwards; | |
background: rgba(16, 16, 16, 0.4); | |
display: flex; | |
flex-direction: column; | |
justify-content: center; | |
align-items: center; | |
border-top-right-radius: 20px; | |
border-bottom-right-radius: 20px; | |
} | |
.box > h2 { | |
color: rgba(195, 195, 195, 0.9); | |
margin-bottom: 30px; | |
} | |
.box .input-box { | |
display: flex; | |
flex-direction: column; | |
box-sizing: border-box; | |
margin-bottom: 10px; | |
} | |
.box .input-box label { | |
font-size: 13px; | |
color: rgba(195, 195, 195, 0.9); | |
margin-bottom: 5px; | |
} | |
.box .input-box input { | |
letter-spacing: 1px; | |
font-size: 14px; | |
box-sizing: border-box; | |
width: 250px; | |
height: 35px; | |
border-radius: 10px; | |
border: 0; | |
background: rgba(195, 195, 195, 0.2); | |
outline: none; | |
padding: 0 12px; | |
color: rgba(195, 195, 195, 0.9); | |
transition: 0.2s; | |
} | |
.box .input-box input:focus { | |
border: 2px solid rgba(195, 195, 195, 0.8); | |
} | |
.box .btn-box { | |
width: 250px; | |
display: flex; | |
flex-direction: column; | |
align-items: start; | |
} | |
.box .btn-box > a { | |
outline: none; | |
display: block; | |
width: 250px; | |
text-align: end; | |
text-decoration: none; | |
font-size: 13px; | |
color: rgba(195, 195, 195, 0.9); | |
} | |
.box .btn-box > a:hover { | |
color: rgba(195, 195, 195, 1); | |
} | |
.box .btn-box > div { | |
margin-top: 10px; | |
display: flex; | |
justify-content: center; | |
align-items: center; | |
} | |
.box .btn-box > button { | |
outline: none; | |
margin-top: 10px; | |
display: block; | |
font-size: 14px; | |
border-radius: 10px; | |
transition: 0.2s; | |
} | |
.box .btn-box > button:nth-of-type(1) { | |
width: 250px; | |
height: 35px; | |
color: rgba(195, 195, 195, 0.9); | |
border: 0; | |
background: rgba(88, 116, 141, 0.5); | |
} | |
.box .btn-box > button:nth-of-type(2) { | |
width: 250px; | |
height: 35px; | |
margin-left: 10px; | |
color: rgba(195, 195, 195, 0.9); | |
border: 0; | |
background: rgba(88, 116, 141, 0.5); | |
} | |
.box .btn-box > button:hover { | |
background: rgba(88, 116, 112, 0.5); | |
border: 3px solid rgba(195, 195, 195, 0.8) | |
} | |
.info_box > button { | |
outline: none; | |
margin: 10px; | |
display: block; | |
font-size: 14px; | |
border-radius: 10px; | |
transition: 0.2s; | |
} | |
.info_box > button:nth-of-type(1) { | |
width: 60px; | |
height: 60px; | |
color: rgba(195,195,195, 0.9); | |
border: 0; | |
background: rgba(41,41,41, 0.5); | |
} | |
.info_box > button:nth-of-type(2) { | |
width: 60px; | |
height: 60px; | |
margin-left: 10px; | |
color: rgba(195, 195, 195, 0.9); | |
border: 0; | |
background: rgba(41,41,41, 0.5); | |
} | |
.info_box > button:hover { | |
background: rgba(110,110,110, 0.5); | |
border: 2px solid rgba(195, 195, 195, 0.8) | |
} | |
p { | |
text-align: center; | |
color: #c3c3c3; | |
font-family: Helvetica, serif; | |
font-size: 100%; | |
display: inline; | |
text-indent: 100px; | |
letter-spacing: 1px; | |
line-height: 120%; | |
} | |
p.warn { | |
text-align: center; | |
color: #e33a3a; | |
font-family: Helvetica, serif; | |
font-size: 100%; | |
display: inline; | |
text-indent: 100px; | |
letter-spacing: 1px; | |
line-height: 120%; | |
} | |
.vl { | |
border-left: 6px solid rgba(5, 5, 5, 0.5); | |
animation: fadeInAnimation ease 1.3s; | |
height: 330px; | |
border-radius: 5px; | |
margin-left: 10px; | |
} | |
::placeholder { | |
color: rgba(41, 41, 41, 0.53); | |
} | |
#overlay { | |
display: block; | |
position: absolute; | |
opacity: 0; | |
top: 0; | |
bottom: 0; | |
background: rgba(16, 16, 16, 0.31); | |
width: 100%; | |
height: 100%; | |
z-index: -1; | |
transition-duration: 0.5s; | |
} | |
#popup { | |
display: block; | |
opacity: 0; | |
position: absolute; | |
top: 50%; | |
left: 50%; | |
width: 500px; | |
height: 500px; | |
margin-left: -250px; /*Half the value of width to center div*/ | |
margin-top: -250px; /*Half the value of height to center div*/ | |
z-index: -1; | |
backdrop-filter: blur(10px); | |
border-radius: 20px; | |
font-family: Arial, sans-serif; | |
color: #c3c3c3; | |
background: rgba(100, 100, 100, 0.3); | |
transition-duration: 0.5s; | |
} | |
#popupclose { | |
float: right; | |
padding: 10px; | |
cursor: pointer; | |
} | |
.popupcontent { | |
padding: 10px; | |
} | |
#button { | |
cursor: pointer; | |
} | |
</style> | |
</head> | |
<body> | |
<div class="box"> | |
<h2>Login</h2> | |
<form method="post"> | |
<div class="input-box"> | |
<label>Account</label> | |
<label><input name="user" type="text" placeholder="Username" oninvalid="this.setCustomValidity('You must enter a username!')" required/></label> | |
</div> | |
<div class="input-box"> | |
<label>Password</label> | |
<label><input name="psw" type="password" placeholder="Password" required/></label> | |
</div> | |
<div class="btn-box"> | |
<a>Forgot password?</a> | |
<button name="Login" type="submit">Login</button> | |
<br> | |
<p class="warn">{{msg}}</p> | |
</div> | |
</form> | |
</div> | |
<div class="vl"></div> | |
<div class="info_box"> | |
<button id="button">About</button> | |
</div> | |
<div id="overlay"></div> | |
<div id="popup"> | |
<div class="popupcontrols"> | |
<span id="popupclose">x</span> | |
</div> | |
<div class="popupcontent"> | |
<h1>About this page</h1> | |
<hr><br> | |
<h2>Theme name</h2> | |
<p>Aero glass</p> | |
<br> | |
<h2>Author</h2> | |
<p>Template: D2Lib<br>Photograph: ArthurZhou - Night of CreeperVillage</p> | |
</div> | |
</div> | |
<script type="text/javascript"> | |
// Initialize Variables | |
var closePopup = document.getElementById("popupclose"); | |
var overlay = document.getElementById("overlay"); | |
var popup = document.getElementById("popup"); | |
var button = document.getElementById("button"); | |
// Close Popup Event | |
closePopup.onclick = function() { | |
overlay.style.opacity = '0'; | |
overlay.style.zIndex = '-1' | |
popup.style.opacity = '0'; | |
popup.style.zIndex = '-1'; | |
}; | |
// Show Overlay and Popup | |
button.onclick = function() { | |
overlay.style.opacity = '1'; | |
overlay.style.zIndex = '100' | |
popup.style.opacity = '1'; | |
popup.style.zIndex = '200'; | |
} | |
</script> | |
</body> | |
</html> |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Before open it, create a folder named
image
at the same directory of this file. Then put a .jpg in the folder and name it asbg.jpg
.Try out suggested photo:
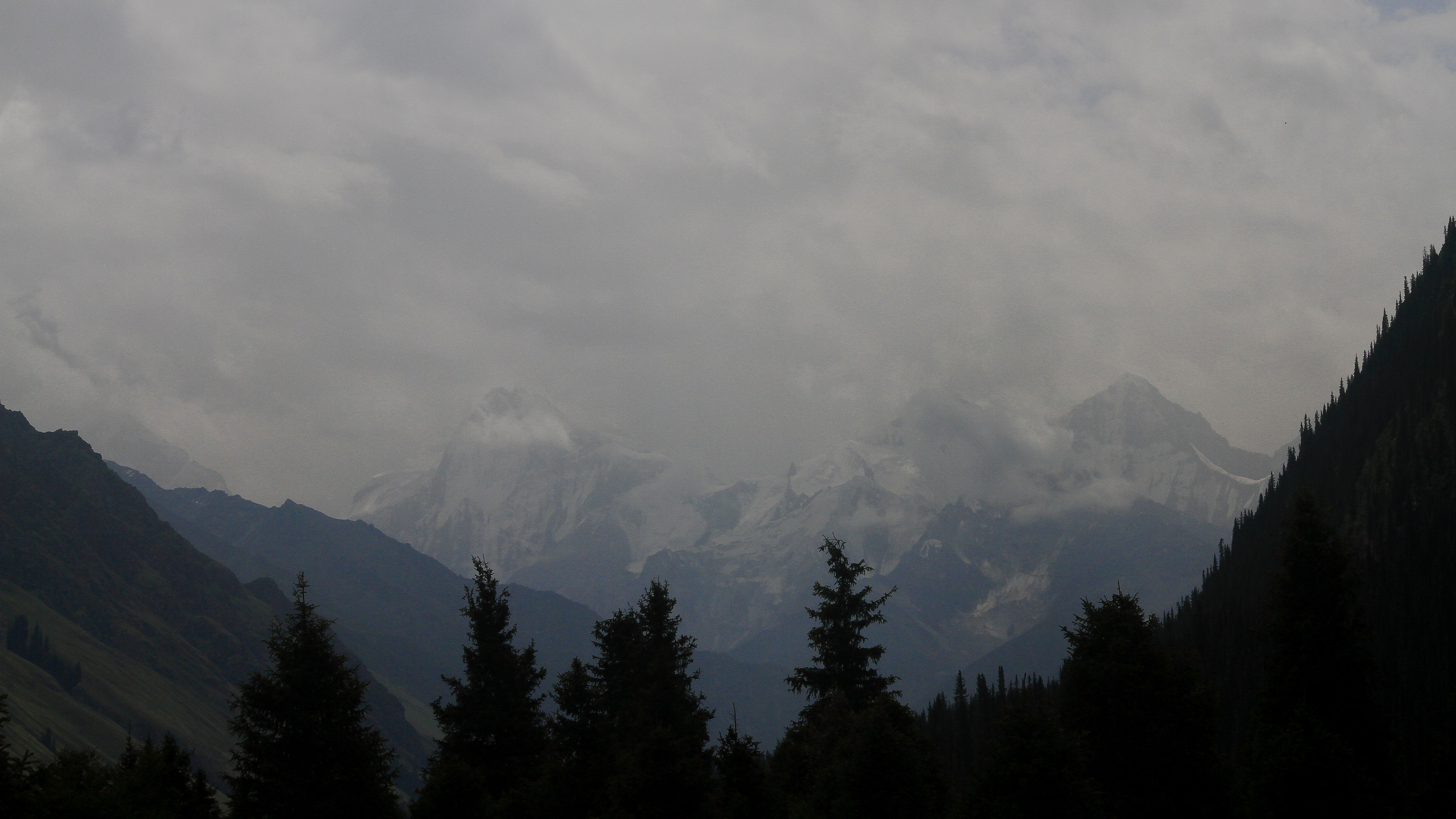